If it won't be simple, it simply won't be. [Hire me, source code] by Miki Tebeka, CEO, 353Solutions
Thursday, May 29, 2008
mikistools
I've placed some of the utilities I use daily at http://code.google.com/p/mikistools/.
Wednesday, May 21, 2008
next_n
Suppose you want to find the next n elements of a stream the matches a predicate.
(I just used it in web scraping with BeautifulSoup to get the next 5 sibling "tr" for a table).
(I just used it in web scraping with BeautifulSoup to get the next 5 sibling "tr" for a table).
#!/usr/bin/env python
from itertools import ifilter, islice
def next_n(items, pred, count):
return islice(ifilter(pred, items), count)
if __name__ == "__main__":
from gmpy import is_prime
from itertools import count
for prime in next_n(count(1), is_prime, 10):
print prime
Will print2(Using gmpy for is_prime)
3
5
7
11
13
17
19
23
29
Thursday, May 15, 2008
Tagcloud
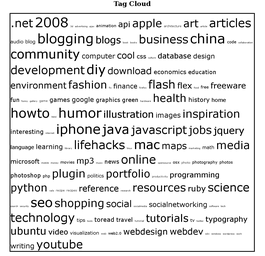
Generating tagcloud (using Mako here, but you can use any other templating system)
tagcloud.cgi
tagcloud.mako
Fading Div
Add a new fading (background) div to your document:
<html>
<body>
</body>
<script>
function set_color(elem) {
var colorstr = elem.fade_color.toString(16).toUpperCase();
/* Pad to 6 digits */
while (colorstr.length < 6) {
colorstr = "0" + colorstr;
}
elem.style.background = "#" + colorstr;
}
function fade(elem, color) {
if (typeof(color) != "undefined") {
elem.fade_color = color;
}
else {
elem.fade_color += 0x001111;
}
set_color(elem);
if (elem.fade_color < 0xFFFFFF) {
setTimeout(function() { fade(elem); }, 200);
}
}
function initialize()
{
var div = document.createElement("div");
div.innerHTML = "I'm Fading";
document.body.appendChild(div);
fade(div, 0xFF0000); /* Red */
}
window.onload = initialize;
</script>
</html>
Subscribe to:
Posts (Atom)